Contents
Introduction:
Hi there, today we will be creating a simple CRUD application. CRUD is an acronym for CREATE, READ, UPDATE, DELETE. The four basic functions in any type of programming.
We will be using Laravel 10. Laravel is a free, open-source PHP Web Framework and intended for the development of web applications following the MVC (Model-View-Controller) architectural pattern. It is designed to make developing web apps faster and easier by using built-in features.
Prerequisite:
- Composer
- MySQL
- PHP >= 8.1
Step 1: Install Laravel 10
First, select a folder where you want Laravel to be installed then execute this command on Terminal or CMD to install Laravel 10:
Install via composer:
composer create-project laravel/laravel:^10.0 laravel-10-crud
Install via Laravel Installer:
laravel new laravel-10-crud
Step 2: Set Database Configuration
After installing, open the .env file and set the database configuration:
.env
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=your database name(laravel_9_crud)
DB_USERNAME=your database username(root)
DB_PASSWORD=your database password(root)
Step 3: Create Model and Database Migration
Model – it is a class that represents a database table.
Migration – like version control for the database that allows us to modify and share database schema to your team.
Execute this command on the Terminal or CMD to create a model and migration:
php artisan make:model Project --migration
After this command, you will find one file in this path “database/migrations” and put the code below on the migration file.
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
return new class extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('projects', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->text('description');
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropIfExists('projects');
}
};
Run the migration by executing the migrate Artisan command:
php artisan migrate
Step 4: Create A Resource Controller
Resource Controller is a controller that handles all HTTP requests stored by your application.
Execute this command to create a resource controller:
php artisan make:controller ProjectController --resource
This command will generate a controller at “app/Http/Controllers/ProjectController.php”. It contains methods for each of the available resource operations. Open the file and insert these codes:
app/Http/Controllers/ProjectController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Models\Project;
class ProjectController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
$projects = Project::get();
return view('projects.index',['projects' => $projects]);
}
/**
* Show the form for creating a new resource.
*
* @return \Illuminate\Http\Response
*/
public function create()
{
return view('projects.create');
}
/**
* Store a newly created resource in storage.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
public function store(Request $request)
{
request()->validate([
'name' => 'required|max:255',
'description' => 'required',
]);
$project = new Project();
$project->name = $request->name;
$project->description = $request->description;
$project->save();
return redirect()->route('projects.index')
->with('success','Project saved successfully!');
}
/**
* Display the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function show($id)
{
$project = Project::find($id);
return view('projects.show', ['project' => $project]);
}
/**
* Show the form for editing the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function edit($id)
{
$project = Project::find($id);
return view('projects.edit', ['project' => $project]);
}
/**
* Update the specified resource in storage.
*
* @param \Illuminate\Http\Request $request
* @param int $id
* @return \Illuminate\Http\Response
*/
public function update(Request $request, $id)
{
request()->validate([
'name' => 'required|max:255',
'description' => 'required',
]);
$project = Project::find($id);
$project->name = $request->name;
$project->description = $request->description;
$project->save();
return redirect()->route('projects.index')
->with('success','Project updated successfully!');
}
/**
* Remove the specified resource from storage.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function destroy($id)
{
Project::destroy($id);
return redirect()->route('projects.index')
->with('success','Project deleted successfully!');
}
}
Step 5: Register a Resourceful Route
After creating a resource controller, register a resourceful route.
routes/web.php
<?php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\ProjectController;
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
|
| Here is where you can register web routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| contains the "web" middleware group. Now create something great!
|
*/
Route::get('/', function () {
return view('welcome');
});
Route::resource('projects', ProjectController::class);
This single route will create multiple routes to handle the CRUD actions on the resource.
Step 6: Create Blade Files
Blade is the simple but powerful templating engine in laravel. blade view files have a .blade.php files extension and are usually stored in “resources/views/” folder.
Create a folder named “projects” inside the following path “resources/views/”. Inside the “projects” folder create the following blade files:
- layout.blade.php
- index.blade.php
- show.blade.php
- create.blade.php
- edit.blade.php
After creating the blade files, Insert the code below:
resources/views/projects/layout.blade.php
<!DOCTYPE html>
<html>
<head>
<title>Laravel Project Manager</title>
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-EVSTQN3/azprG1Anm3QDgpJLIm9Nao0Yz1ztcQTwFspd3yD65VohhpuuCOmLASjC" crossorigin="anonymous">
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/js/bootstrap.bundle.min.js" integrity="sha384-MrcW6ZMFYlzcLA8Nl+NtUVF0sA7MsXsP1UyJoMp4YLEuNSfAP+JcXn/tWtIaxVXM" crossorigin="anonymous"></script>
</head>
<body>
<div class="container">
@yield('content')
</div>
</body>
</html>
resources/views/projects/index.blade.php
@extends('projects.layout')
@section('content')
<div class="container">
<h2 class="text-center mt-5 mb-3">Laravel Project Manager</h2>
<div class="card">
<div class="card-header">
<a class="btn btn-outline-primary" href="{{ route('projects.create') }}">
Create New Project
</a>
</div>
<div class="card-body">
@if ($message = Session::get('success'))
<div class="alert alert-success">
<b>{{ $message }}</b>
</div>
@endif
<table class="table table-bordered">
<tr>
<th>Name</th>
<th>Description</th>
<th width="240px">Action</th>
</tr>
@foreach ($projects as $project)
<tr>
<td>{{ $project->name }}</td>
<td>{{ $project->description }}</td>
<td>
<form action="{{ route('projects.destroy',$project->id) }}" method="POST">
<a
class="btn btn-outline-info"
href="{{ route('projects.show',$project->id) }}">
Show
</a>
<a
class="btn btn-outline-success"
href="{{ route('projects.edit',$project->id) }}">
Edit
</a>
@csrf
@method('DELETE')
<button type="submit" class="btn btn-outline-danger">Delete</button>
</form>
</td>
</tr>
@endforeach
</table>
</div>
</div>
</div>
@endsection
resources/views/projects/show.blade.php
@extends('projects.layout')
@section('content')
<div class="container">
<h2 class="text-center mt-5 mb-3">Show Project</h2>
<div class="card">
<div class="card-header">
<a class="btn btn-outline-info float-right" href="{{ route('projects.index') }}">
View All Projects
</a>
</div>
<div class="card-body">
<b class="text-muted">Name:</b>
<p>{{$project->name}}</p>
<b class="text-muted">Description:</b>
<p>{{$project->description}}</p>
</div>
</div>
</div>
@endsection
resources/views/projects/create.blade.php
@extends('projects.layout')
@section('content')
<div class="container">
<h2 class="text-center mt-5 mb-3">Create New Project</h2>
<div class="card">
<div class="card-header">
<a class="btn btn-outline-info float-right" href="{{ route('projects.index') }}">
View All Projects
</a>
</div>
<div class="card-body">
@if ($errors->any())
<div class="alert alert-danger">
<ul>
@foreach ($errors->all() as $error)
<li>{{ $error }}</li>
@endforeach
</ul>
</div>
@endif
<form action="{{ route('projects.store') }}" method="POST">
@csrf
<div class="form-group">
<label for="name">Name</label>
<input type="text" class="form-control" id="name" name="name">
</div>
<div class="form-group">
<label for="description">Description</label>
<textarea class="form-control" id="description" rows="3" name="description"></textarea>
</div>
<button type="submit" class="btn btn-outline-primary mt-3">Save Project</button>
</form>
</div>
</div>
</div>
@endsection
resources/views/projects/edit.blade.php
@extends('projects.layout')
@section('content')
<div class="container">
<h2 class="text-center mt-5 mb-3">Edit Project</h2>
<div class="card">
<div class="card-header">
<a class="btn btn-outline-info float-right" href="{{ route('projects.index') }}">
View All Projects
</a>
</div>
<div class="card-body">
@if ($errors->any())
<div class="alert alert-danger">
<ul>
@foreach ($errors->all() as $error)
<li>{{ $error }}</li>
@endforeach
</ul>
</div>
@endif
<form action="{{ route('projects.update',$project->id) }}" method="POST">
@csrf
@method('PUT')
<div class="form-group">
<label for="name">Name</label>
<input type="text" class="form-control" id="name" name="name" value="{{ $project->name }}">
</div>
<div class="form-group">
<label for="description">Description</label>
<textarea class="form-control" id="description" rows="3" name="description">{{ $project->description }}</textarea>
</div>
<button type="submit" class="btn btn-outline-success mt-3">Save Project</button>
</form>
</div>
</div>
</div>
@endsection
Step 7: Run the Application
After finishing the steps above, you can now run your application by executing the code below:
php artisan serve
After successfully running your app, open this URL in your browser:
http://127.0.0.1:8000/projects
Screenshots:
Laravel 10 Crud App (project index page):
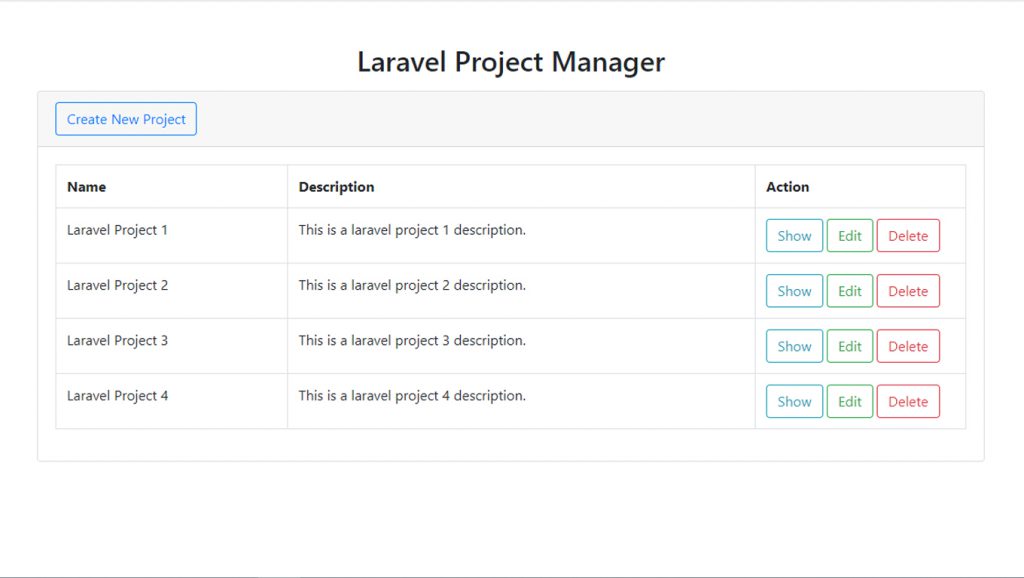
Laravel 10 Crud App (project create page):
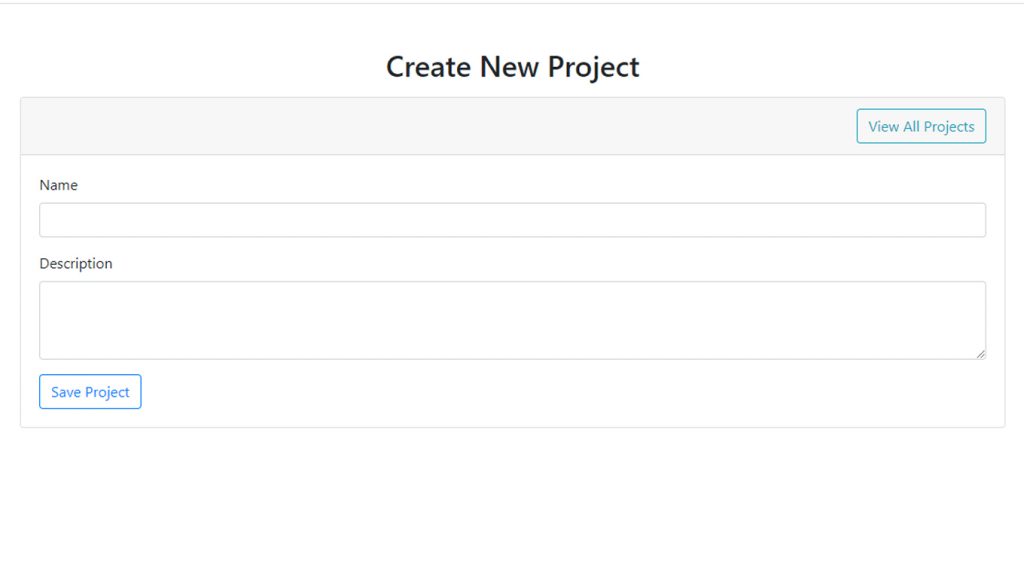
Laravel 10 Crud App (project edit page):
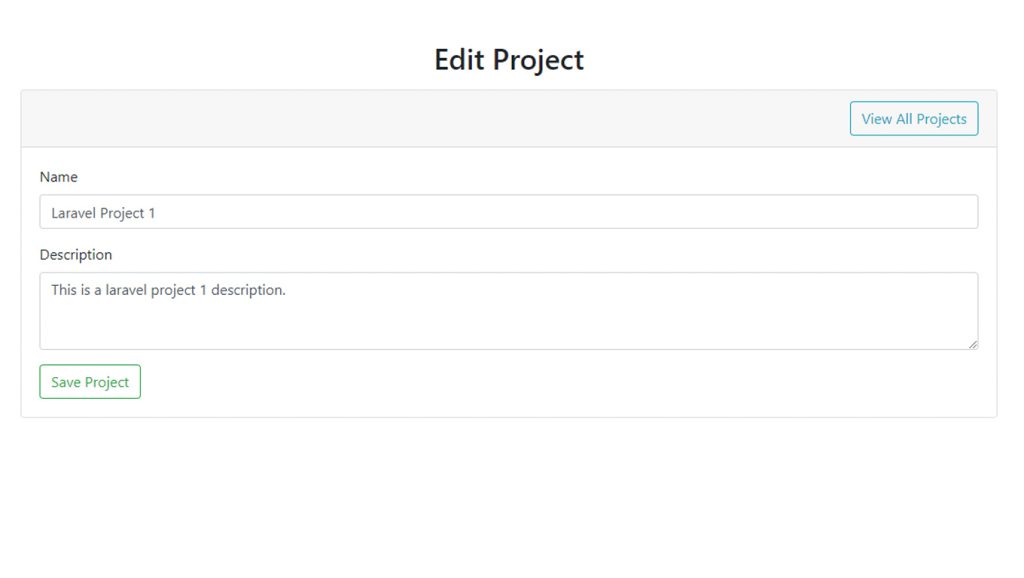
Laravel 10 Crud App (project show page):
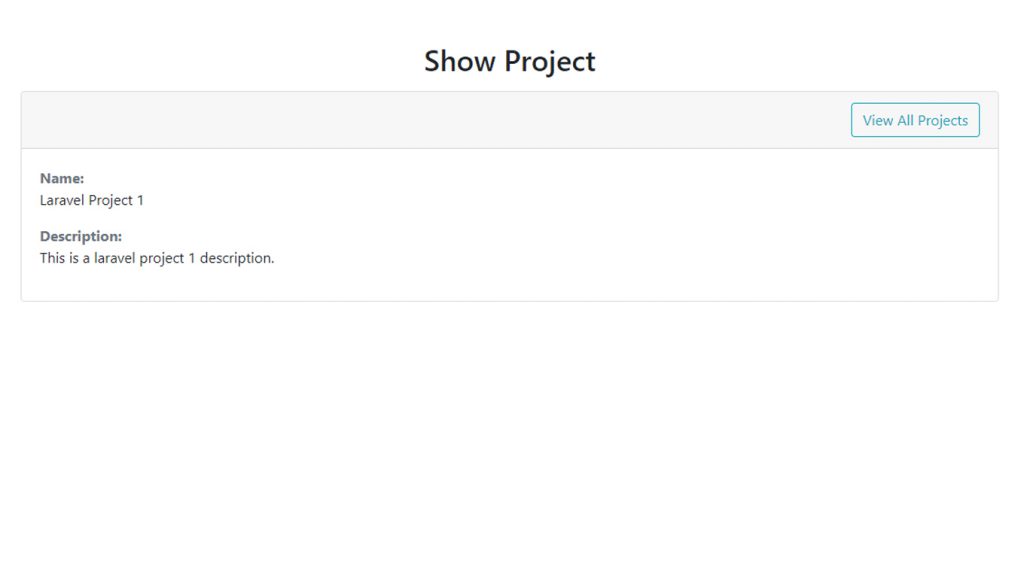